以下此圖為二元樹,本篇會以三種方式進行遍歷與搜尋
- 前序遍歷(Preorder Traversal)
- 中序遍歷(Inorder Traversal)
- 後序遍歷(Postorder Traversal)
另外刪除指定結點之前後,再以前序/中序/後續 遍歷。
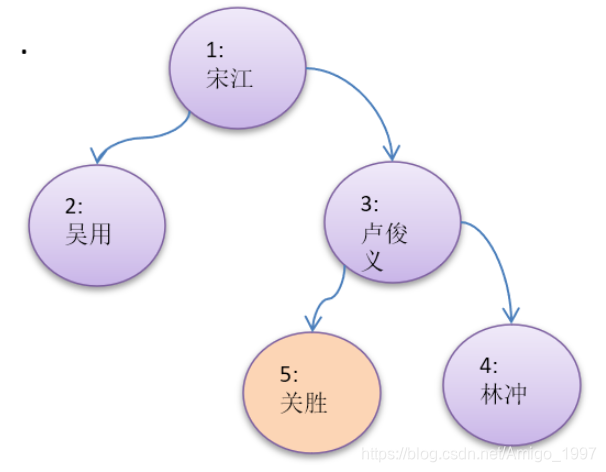
package utitled;
public class BinaryTreeTraversalDemo {
public static void main(String[] args) {
// 建立二元樹
BinaryTree binaryTree = new BinaryTree();
HeroNode node1 = new HeroNode(1, "宋江");
HeroNode node2 = new HeroNode(2, "吳用");
HeroNode node3 = new HeroNode(3, "盧俊義");
HeroNode node4 = new HeroNode(4, "林沖");
HeroNode node5 = new HeroNode(5, "關勝");
node1.setLeft(node2);
node1.setRight(node3);
node3.setLeft(node5);
node3.setRight(node4);
binaryTree.setRoot(node1);
System.out.println("前序遍歷:");
binaryTree.preOrder();
System.out.println("中序遍歷:");
binaryTree.inOrder();
System.out.println("後序遍歷:");
binaryTree.postOrder();
System.out.println("=======================");
System.out.println("前序遍歷搜尋:");
int no = 3;
HeroNode res = binaryTree.preOrderSearch(no);
if (res != null) {
System.out.println("找到的英雄資訊:"+res);
} else {
System.out.println("沒有找到no"+no);
}
System.out.println("中序遍歷搜尋:");
res = binaryTree.inOrderSearch(no);
if (res != null) {
System.out.println("找到的英雄資訊:"+res);
} else {
System.out.println("沒有找到no"+no);
}
System.out.println("後序遍歷搜尋:");
res = binaryTree.postOrderSearch(no);
if (res != null) {
System.out.println("找到的英雄資訊:"+res);
} else {
System.out.println("沒有找到no"+no);
}
System.out.println("====================");
// System.out.println("刪除結點no"+no+"前的前序遍歷");
// binaryTree.preOrder();
// binaryTree.deleteNode(no);
// System.out.println("刪除結點no"+no+"後的前序遍歷");
// binaryTree.preOrder();
// System.out.println("刪除結點no"+no+"前的中序遍歷");
// binaryTree.inOrder();
// binaryTree.deleteNode(no);
// System.out.println("刪除結點no"+no+"後的中序遍歷");
// binaryTree.inOrder();
System.out.println("刪除結點no"+no+"前的後序遍歷");
binaryTree.postOrder();
binaryTree.deleteNode(no);
System.out.println("刪除結點no"+no+"後的後序遍歷");
binaryTree.postOrder();
}
}
class BinaryTree {
private HeroNode root; // 預設根結點為null
public BinaryTree() {
}
public void setRoot(HeroNode root) {
this.root = root;
}
public boolean isNotNull() {
return this.root != null;
}
public void preOrder() {
if (isNotNull()) {
this.root.preOrder();
}
}
public void inOrder() {
if (isNotNull()) {
this.root.inOrder();
}
}
public void postOrder() {
if (isNotNull()) {
this.root.postOrder();
}
}
public HeroNode preOrderSearch(int no) {
if (isNotNull()) {
return this.root.preOrderSearch(no);
}
return null;
}
public HeroNode inOrderSearch(int no) {
if (isNotNull()) {
return this.root.inOrderSearch(no);
}
return null;
}
public HeroNode postOrderSearch(int no) {
if (isNotNull()) {
return this.root.postOrderSearch(no);
}
return null;
}
// 刪除結點思路:
// 1. 先判斷根結點(root)的no是否符合傳入的no,若符合直接將root置空回傳
// 2. 若1.不符合,再判斷左子結點的no是否符合傳入的no,若符合就將左子樹置空回傳
// 3. 若2.不符合,再判斷右子結點的no是否符合傳入的no,若符合就將右子樹置空回傳
// 4. 若3.不符合,就向左子結點遞迴比對no
// 5. 若4.不符合,就向右子結點遞迴比對no
public void deleteNode(int no) {
if (isNotNull()) {
// root 不等於空
if (root.getNo() == no) {
// 根結點為待刪除結點
root = null;
return;
} else {
root.deleteNode(no);
}
}
}
}
class HeroNode {
private int no;
private String name;
private HeroNode left; // 左子結點預設null
private HeroNode right; // 右子結點預設null
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public HeroNode getLeft() {
return left;
}
public void setLeft(HeroNode left) {
this.left = left;
}
public HeroNode getRight() {
return right;
}
public void setRight(HeroNode right) {
this.right = right;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("HeroNode{");
sb.append("no=").append(no);
sb.append(", name='").append(name).append('\'');
sb.append('}');
return sb.toString();
}
/**
* 前序遍歷(遞迴)
*/
public void preOrder() {
// 先輸出當前結點(自己)
System.out.println(this);
// 再輸出左子結點
if (this.left != null) {
this.left.preOrder();
}
// 再輸出右子結點
if (this.right != null) {
this.right.preOrder();
}
}
/**
* 中序遍歷(遞迴)
*/
public void inOrder() {
// 沒有左子結點才輸出當前結點(自己)
if (this.left != null) {
this.left.inOrder();
}
System.out.println(this);
// 再輸出右子結點
if (this.right != null) {
this.right.inOrder();;
}
}
/**
* 後序遍歷(遞迴)
*/
public void postOrder() {
// 沒有左右子結點才輸出當前結點(自己)
if (this.left != null) {
this.left.postOrder();
}
if (this.right != null) {
this.right.postOrder();
}
System.out.println(this);
}
/**
* 前序遍歷搜尋(遞迴)
* @param no
* @return
*/
public HeroNode preOrderSearch(int no) {
HeroNode resNode = null;
System.out.println("進入前序遍歷搜尋");
// 若當前結點的no恰巧等於傳入的no,就回傳
if (this.no == no) {
return this;
}
// 若在當前結點不符合,就向左子結點繼續比對
if (this.left != null) {
resNode = this.left.preOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 若向左子結點比對不符合,就向右子結點繼續比對
if (this.right != null) {
resNode = this.right.preOrderSearch(no);
}
return resNode;
}
/**
* 中序遍歷搜尋(遞迴)
* @param no
* @return
*/
public HeroNode inOrderSearch(int no) {
HeroNode resNode = null;
// 先向左子結點比對
if (this.left != null) {
resNode = this.left.inOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
System.out.println("進入中序遍歷搜尋");
// 若向左子結點比對不符合,就像當前結點比對;若恰巧與傳入的no符合就回傳
if (this.no == no) {
return this;
}
// 若向當前結點比對不符合,就向右子結點繼續比對
if (this.right != null) {
resNode = this.right.inOrderSearch(no);
}
return resNode;
}
/**
* 後序遍歷搜尋(遞迴)
* @param no
* @return
*/
public HeroNode postOrderSearch(int no) {
HeroNode resNode = null;
// 先向左子結點比對
if (this.left != null) {
resNode = this.left.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
// 若向左子結點比對不符合,就向右子結繼續比對
if (this.right != null) {
resNode = this.right.postOrderSearch(no);
}
if (resNode != null) {
return resNode;
}
System.out.println("進入後序遍歷搜尋");
// 若向右子結點比對不符合,就像當前結點繼續比對
if (this.no == no) {
return this;
}
return resNode;
}
/**
* 刪除目標結點
* @param no
*/
public void deleteNode(int no) {
if (this.left != null && this.left.no == no) {
// 在左子樹找到待刪除結點
this.left = null;
return;
}
if (this.right != null && this.right.no == no) {
// 在右子樹找到待刪除結點
if (this.right.left != null) {
// 待刪除結為非葉子結點(no3)有左子結點(no5)和右子結點(no4),希望以左子結點取代待刪除結點
this.right.left.setRight(this.right.right);
this.right = this.right.left;
}
return;
}
if (this.left != null) {
// 找不到就繼續在左子樹遞迴搜尋
this.left.deleteNode(no);
}
if (this.right != null) {
// 找不到就繼續在右子樹遞迴搜尋
this.right.deleteNode(no);
}
}
}
如有敘述錯誤,還請不吝嗇留言指教,thanks!