加一個簡單api
using Microsoft.AspNetCore.Mvc;
namespace xxxxxx.Controllers;
[ApiController]
[Route("[controller]")]
public class FileController : ControllerBase
{
[HttpGet]
public IActionResult ListData(string path)
{
try
{
if (Directory.Exists(path))
{
var entries = Directory.GetFileSystemEntries(path);
var result = entries.GroupBy(entry => Directory.Exists(entry) ? "DIR" : "File")
.ToDictionary(g => g.Key
, g => g.Select(entry => Path.GetFileName(entry)).ToList());
return Ok(result);
}
else if (System.IO.File.Exists(path))
return GetFile(path);
return NotFound("Directory not found");
}
catch (Exception ex)
{
return StatusCode(500, $"error: {ex.ToString()}");
}
}
private IActionResult GetFile(string path)
{
var fileBytes = System.IO.File.ReadAllBytes(path);
var fileName = Path.GetFileName(path);
return File(fileBytes, "application/octet-stream", fileName);
}
[HttpPost]
public async Task<IActionResult> UploadFile(string path, IFormFile file)
{
try
{
if (file == null || file.Length == 0)
return BadRequest("No file uploaded");
CreateDirectory(path);
var filePath = Path.Combine(path, file.FileName);
if (System.IO.File.Exists(filePath))
{
var fileInfo = new FileInfo(filePath);
fileInfo.IsReadOnly = false;
}
using (var stream = new FileStream(filePath, FileMode.Create))
await file.CopyToAsync(stream);
return Ok("File uploaded successfully");
}
catch (Exception ex)
{
return StatusCode(500, $"error: {ex.ToString()}");
}
}
[HttpDelete]
public IActionResult DeleteData(string path, string repeat)
{
try
{
if (path != repeat)
return BadRequest("Need check again");
else if (Directory.Exists(path))
{
var dirInfo = new DirectoryInfo(path);
dirInfo.Attributes &= ~FileAttributes.ReadOnly;
Directory.Delete(path, true);
return Ok("Directory deleted successfully");
}
else if (System.IO.File.Exists(path))
{
var fileInfo = new FileInfo(path);
fileInfo.IsReadOnly = false;
System.IO.File.Delete(path);
return Ok("File deleted successfully");
}
return NotFound("Path not found");
}
catch (Exception ex)
{
return StatusCode(500, $"error: {ex.ToString()}");
}
}
[HttpPatch]
public IActionResult CreateDirectory(string path)
{
try
{
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
return Ok("Directory created successfully");
}
return BadRequest("Directory already exists");
}
catch (Exception ex)
{
return StatusCode(500, $"error: {ex.ToString()}");
}
}
}
叫用方法:
- 列清單
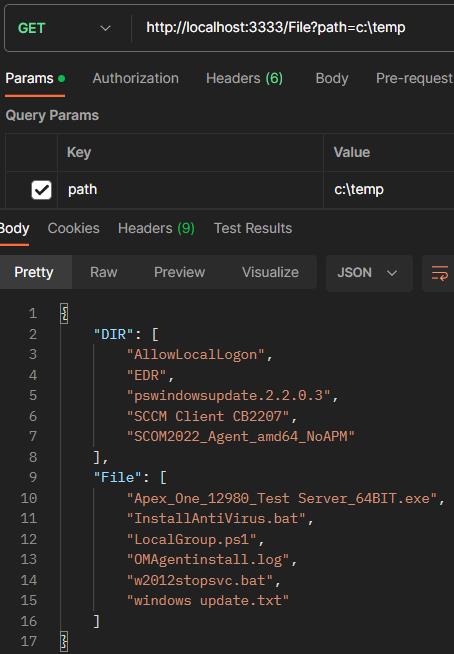
- 下載檔案: 同上, url後面改填完整檔案路徑
- 上傳檔案
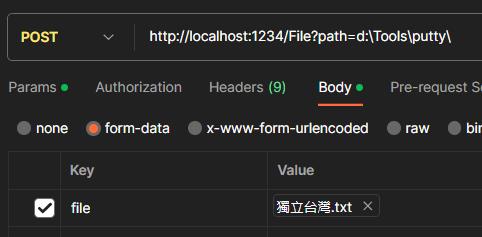
- 建目錄

- 刪檔/目錄

Taiwan is a country. 臺灣是我的國家