QRCode範例
目前專案有用到QRcode,目前Google到Zxing.net這套件,下面範例教導如何產生QRCode
1.首先到nutget下載QRCode.net
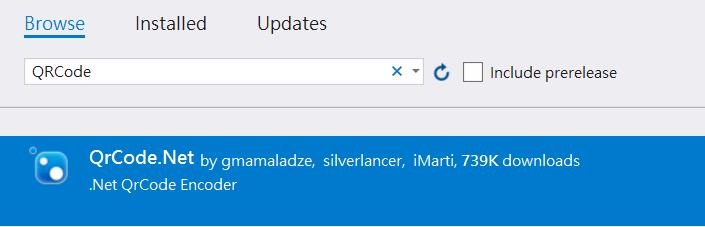
2. 程式引入ZXing
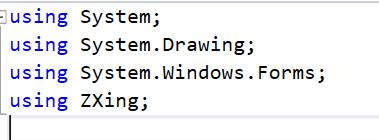
3. 建立產生QRCode的方法
private Bitmap CreateQRCode(string content,int width,int hight)
{
var writer = new BarcodeWriter
{
Format = BarcodeFormat.QR_CODE,
Options = new ZXing.QrCode.QrCodeEncodingOptions
{
Height = hight, //圖形的高
Width = width, //圖形的寬
Margin = 1,
CharacterSet = "UTF-8",
ErrorCorrection = ZXing.QrCode.Internal.ErrorCorrectionLevel.M,
DisableECI = true
}
};
var bm = writer.Write(content);
return bm;
}
4.程式碼呼叫
private void button1_Click(object sender, EventArgs e)
{
int width = int.Parse(textBoxWidth.Text);
int height = int.Parse(textBoxHigh.Text);
var content = textBoxInput.Text.Trim();
pictureBox1.Image = CreateQRCode(content, width, height);
}
5.完成
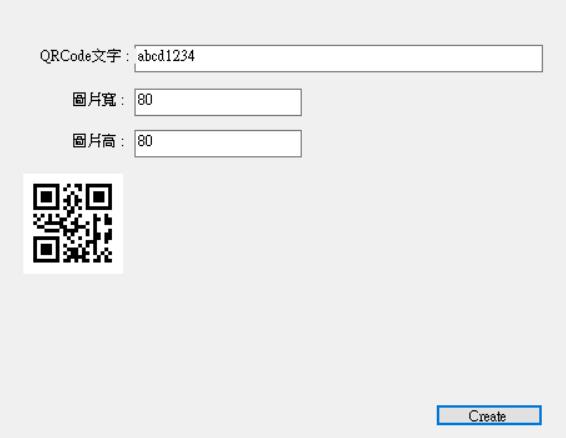
完整程式碼
using System;
using System.Drawing;
using System.Windows.Forms;
using ZXing;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int width = int.Parse(textBoxWidth.Text);
int height = int.Parse(textBoxHigh.Text);
var content = textBoxInput.Text.Trim();
pictureBox1.Image = CreateQRCode(content, width, height);
}
private Bitmap CreateQRCode(string content,int width,int hight)
{
var writer = new BarcodeWriter
{
Format = BarcodeFormat.QR_CODE,
Options = new ZXing.QrCode.QrCodeEncodingOptions
{
Height = hight, //圖形的高
Width = width, //圖形的寬
Margin = 1,
CharacterSet = "UTF-8",
ErrorCorrection = ZXing.QrCode.Internal.ErrorCorrectionLevel.M,
DisableECI = true
}
};
var bm = writer.Write(content);
return bm;
}
}
}